LiveCode is the premier environment for creating multi-platform solutions for all major operating systems - Windows, Mac OS X, Linux, the Web, Server environments and Mobile platforms. Brand new to LiveCode? Welcome!
Moderators: FourthWorld, heatherlaine, Klaus, kevinmiller, robinmiller
-
trevix
- Posts: 962
- Joined: Sat Feb 24, 2007 11:25 pm
- Location: Italy
-
Contact:
Post
by trevix » Tue Sep 05, 2023 10:37 am
Hello.
I am trying to find the best and fastest way, on standalone opening, to delete stored files, according to some requirements:
I have a list of file names (there may be hundreds of lines) similar to this (underscore delimited):
I would like to only keep the newest first 10 files "for each user email". That is, to get a list of files to delete from the storage so that what is left are the most recent 10 files for each user.
I could do it with a lots of repeat loop but I think there could be a better way using arrays.
Any idea?
Thanks
Trevix
Trevix
OSX 14.3.1 xCode 15 LC 10 DP7 iOS 15> Android 7>
-
Klaus
- Posts: 13829
- Joined: Sat Apr 08, 2006 8:41 am
- Location: Germany
-
Contact:
Post
by Klaus » Tue Sep 05, 2023 10:59 am
Buongiorno trevix,
arrays? Come on, no need for arrays!
1. Get the list of -> the detailed files
2. Sort this list by item 5 (Last modification date)
3. Delete line 1 to 10 of this list
4. Now use a repeat loop through the rest of the list and delete all these files.
Or did I misunderstand your problem?
Best
Klaus
-
trevix
- Posts: 962
- Joined: Sat Feb 24, 2007 11:25 pm
- Location: Italy
-
Contact:
Post
by trevix » Tue Sep 05, 2023 11:20 am
The best I could think of, is the following:
Code: Select all
on mouseUp
--create a dummy list
repeat with U = 1 to 11
put U & "_testuser2@" & cr after tList
end repeat
put "12_testuser1@" & cr after tList
put "cane_testuser3@" after tList
--get list of owners
set the itemdelimiter to "_"
repeat for each line tLine in tList
put empty into tOwnersArray[item 2 of tLine][tLine]
end repeat
breakpoint
repeat for each line tEmail in the keys of tOwnersArray
put the keys of tOwnersArray[tEmail] into tOwnerList
sort lines of tOwnerList descending numeric by item 1 of each
repeat with U = 11 to the number of lines of tOwnerList
put line U of tOwnerList & cr after tDeleteList
end repeat
end repeat
breakpoint
end mouseUp
Only 1_testuser2@ gets deleted, the older one of the 11 files of testuser2@
Trevix
OSX 14.3.1 xCode 15 LC 10 DP7 iOS 15> Android 7>
-
LCMark
- Livecode Staff Member
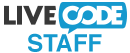
- Posts: 1209
- Joined: Thu Apr 11, 2013 11:27 am
Post
by LCMark » Tue Sep 05, 2023 11:39 am
@trevix: Assuming these files all sit in their own folder then you can do:
Code: Select all
command keepRecentFiles pFolder, pCount
/* Fetch the list of all files in the specified folder. */
local tFiles
put files(pFolder) into tFiles
/* The filenames are of the form YYYMMDDHHMMSS_<email>. So set the
* the item delimiter appropriately to make accessing the timestamp
* easy, and then sort descending by the first item so the most recent
* files are first. */
set the itemDelimiter to "_"
sort tFiles descending text by item 1 of each
set the itemDelimiter to comma
/* Loop through the files, skipping the first pCount, and
* deleting the rest. */
local tIndex
repeat for each line tFile in tFiles
add 1 to tIndex
if tIndex <= pCount then
next repeat
end if
delete file (pFolder & slash & tFile)
end repeat
end keepRecentFiles
Note: The sort is 'text' because timestamps which are YYYYMMDDHHMMSS have the same order as both numbers and text, but by using text you avoid any numeric conversions.
-
Klaus
- Posts: 13829
- Joined: Sat Apr 08, 2006 8:41 am
- Location: Germany
-
Contact:
Post
by Klaus » Tue Sep 05, 2023 11:44 am
Oops, sorry. completely overlooked -> "for each user email"
-
LCMark
- Livecode Staff Member
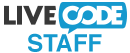
- Posts: 1209
- Joined: Thu Apr 11, 2013 11:27 am
Post
by LCMark » Tue Sep 05, 2023 12:37 pm
@Klaus: You aren't the only one

-
jacque
- VIP Livecode Opensource Backer
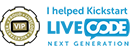
- Posts: 7239
- Joined: Sat Apr 08, 2006 8:31 pm
- Location: Minneapolis MN
-
Contact:
Post
by jacque » Tue Sep 05, 2023 6:23 pm
@trevix, your handler should work if you change this line:
Code: Select all
repeat with U = 11 to the number of lines of tOwnerList
to this:
Code: Select all
repeat with U = the number of lines of tOwnerList down to 11
When deleting items or lines in a list you have to delete backwards. Otherwise what used to be line 12 becomes line 11 after the first deletion finishes.
Jacqueline Landman Gay | jacque at hyperactivesw dot com
HyperActive Software | http://www.hyperactivesw.com
-
trevix
- Posts: 962
- Joined: Sat Feb 24, 2007 11:25 pm
- Location: Italy
-
Contact:
Post
by trevix » Tue Sep 05, 2023 6:41 pm
Correct, Jacque. Thanks.
Any way I was hoping on some slicker trick to do this thing.
All these repeat loop may not be efficient enough when deleting from a list of hundreds filenames.
One more thing that I don't know if the "delete file filepath" command is time consuming or not.
My final code is the following:
Code: Select all
--create a dummy list
repeat with U = 1 to 11
put U & "_testuser2@" & cr after tList
end repeat
put "12_testuser1@" & cr after tList
put "cane_testuser3@" after tList
--get list of owners
set the itemdelimiter to "_"
repeat for each line tLine in tList
put empty into tOwnersArray[item 4 of tLine][tLine]
end repeat
repeat for each line tEmail in the keys of tOwnersArray
put the keys of tOwnersArray[tEmail] into tOwnerList
sort lines of tOwnerList descending text by item 1 of each --use text for yyyymmddhhmm
repeat with U = 11 to the number of lines of tOwnerList
put line U of tOwnerList into tFile
delete file gPrefTF["AllPath"]["PathBackup" & gSport] & tFile
end repeat
end repeat
Trevix
OSX 14.3.1 xCode 15 LC 10 DP7 iOS 15> Android 7>
-
jacque
- VIP Livecode Opensource Backer
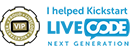
- Posts: 7239
- Joined: Sat Apr 08, 2006 8:31 pm
- Location: Minneapolis MN
-
Contact:
Post
by jacque » Wed Sep 06, 2023 5:43 pm
The best way to know how fast deletions will be is to just time it on some sample data, but in general I LC is very fast when working with files and unless you have thousands of files I don't think there will be too much delay.
Jacqueline Landman Gay | jacque at hyperactivesw dot com
HyperActive Software | http://www.hyperactivesw.com